RSS stands for Really Simple Syndication. It’s an XML-based content format for distributing content, news etc. It makes your content global and available to various platforms. Most popular sites and blogs provide RSS feeds for you to subscribe. You need a feed reader to view its contents, that’s all. You can also create RSS feeds for your own website. In this post I am explaining how to create RSS feed in PHP.
Let’s assume you have an array of items like below:
$items = array( array( "title" => "First Item", "description" => "First item description", "link" => "http://www.tricksofit.com/2013/10/seo-tips-tricks" ), array( "title" => "Second Item", "description" => "Second item description", "link" => "http://www.tricksofit.com/2013/11/how-to-use-cookies-in-javascript" ), array( "title" => "Third Item", "description" => "Third item description", "link" => "http://www.tricksofit.com/2013/12/generate-and-implement-simple-captcha-code-in-php" ) );
Basic Structure of RSS feed is look like as:
<?xml version="1.0" encoding="utf-8"?> <rss version="2.0"> <channel> <title>Website title</title> <link>Website's url</link> <description>Describe your website/feed</description> <language>en-us</language> <item> <title>Your item title</title> <description>Your item description</description> <link>item url</link> </item> <item> ... ... </item> </channel> </rss>
Now to create RSS feed you need to tell the browser that your are presenting RSS feed, then create SimpleXMLElement object and set RSS version 2.0 for RSS reader like below:
header("Content-Type: application/rss+xml; charset=UTF-8"); $xml = new SimpleXMLElement('<rss/>'); $xml->addAttribute("version", "2.0");
As in basic structure the RSS feed contains an element ‘channel’ tag which contains all information about item, which are stored in elements ‘item’ tag. First, we’ll add the basic information to the feed:
$channel = $xml->addChild("channel"); $channel->addChild("title", "Website title"); $channel->addChild("link", "Website's url"); $channel->addChild("description", "Describe your website/feed"); $channel->addChild("language", "en-us");
Now we need to add item from the array of items to this feed like as below:
foreach ($items as $row) { $item = $channel->addChild("item"); $item->addChild("title", $row['title']); $item->addChild("link", $row['link']); $item->addChild("description", $row['description']); }
Now feed is ready, you can see the output of the feed using echo like:
echo $xml->asXML();
These are thee simple steps for how to create RSS feed in php. Now lets discuss the advantages of RSS feeds.
Advantages of RSS Feeds:
RSS feeds is an excellent way to increase traffic to your site. It makes your content global and available to various platforms to use.
Sometimes when you send an email newsletter more than 30-40% of the people won’t even receive it due to spam filters or it gets in the junk mail. But with RSS feeds your are sending your content for all the readers, no need to send email newsletters.
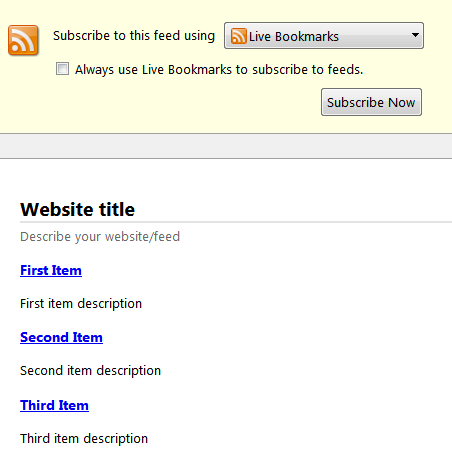