Regular expression offers an extremely flexible and powerful way of validation for website forms. Regular Expression with jQuery validation ensure you that provided user data is as per our requirements. In this tutorial I am describing some example Regular Expression with jQuery Validation.
Regular Expression for Email:
To validate the email address I have created a function based on standard regular expression which returns true or false. You just need to pass the email to the function checkEmail(email).
//function used to check valid email function checkEmail(email) { //regular expression for email var pattern = new RegExp(/^(("[\w-\s]+")|([\w-]+(?:\.[\w-]+)*)|("[\w-\s]+")([\w-]+(?:\.[\w-]+)*))(@((?:[\w-]+\.)*\w[\w-]{0,66})\.([a-z]{2,6}(?:\.[a-z]{2})?)$)|(@\[?((25[0-5]\.|2[0-4][0-9]\.|1[0-9]{2}\.|[0-9]{1,2}\.))((25[0-5]|2[0-4][0-9]|1[0-9]{2}|[0-9]{1,2})\.){2}(25[0-5]|2[0-4][0-9]|1[0-9]{2}|[0-9]{1,2})\]?$)/i); if(pattern.test(email)){ return true; } else { return false; } }
You can use following simple regular expression for email validation.
var pattern = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$/;
Result:
user.email.com = Error
user@email.com = Ok
Regular Expression for URL:
To validate the URL I have created a function that accept http or https with URL and returns true or false. You need to pass the URL to checkUrl(url).
function checkUrl(url) { //regular expression for URL var pattern = /^(http|https)?:\/\/[a-zA-Z0-9-\.]+\.[a-z]{2,4}/; if(pattern.test(url)){ return true; } else { return false; } }
Result:
www.tricksofit.com = Error
http://tricksofit.com = Ok
http://www.tricksofit.com = Ok
Regular Expression for Username:
To validate a username I have created a function that accept 4-15 characters without any special characters. You can pass the username value to checkUsername(username).
function checkUsername(username){ var pattern = /^[a-z0-9_-]{4,15}$/; if(pattern.test(username)){ return true; }else{ return false; } }
Result:
user.name = Error
username123 = Ok
Regular Expression for Password:
To validate a password I have created a function that accept 5-15 characters, lowercase, uppercase, numbers. You can pass the password value to checkPassword(password).
function checkPassword(password){ var pattern = /^[a-zA-Z0-9_-]{5,15}$/; if(pattern.test(password)){ return true; }else{ return false; } }
Result:
myPass = Ok
my@Pass = Error
Regular Expression for Strong Password:
To validate a strong password you can use following regular expression that allows:
- Must be at least 8 characters
- At least 1 number, 1 lowercase, 1 uppercase letter
- At least 1 special character from @#$%&
var pattern = /^.*(?=.{8,})(?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.*[@#$%&]).*$/;
Result:
myPass@123 = Ok
my@Pass = Error
Conclusion:
You can use above regular expressions and modify as you like. To understand the regular expression and create a new regular expression you can follow the instruction from the reference.
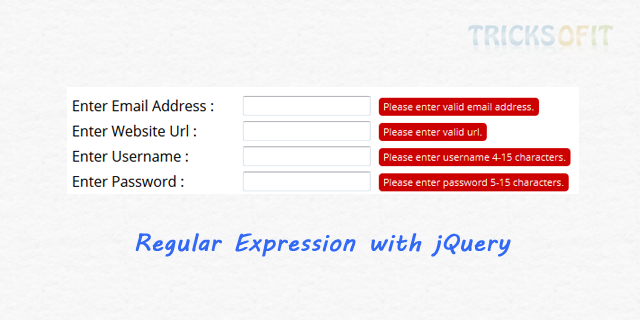