Sometimes It is often handy to have a proper method to get number of days and number of months between two dates. In this article, I am discussing how to calculate the difference between two dates in PHP.
Calculate months between two dates:
For PHP >=5.3 you can use DateTime diff that returns a DateInterval object as below.
$d1 = new DateTime("2013-12-09"); $d2 = new DateTime("2014-03-17"); var_dump($d1->diff($d2)->m); // int(3) var_dump($d1->diff($d2)->m + ($d1->diff($d2)->y*12)); // int(3)
If you don’t have PHP 5.3 or higher, you can use strtotime() function to get timestamps, the number of seconds between any date and January 1 1970 00:00:00.
$d1 = "2013-12-09"; $d2 = "2014-03-17"; echo (int)abs((strtotime($d1) - strtotime($d2))/(60*60*24*30)); // 3
The PHP ABS() absolute value to always return a positive number as the number of months between the two dates.
But it’s not very precise because there is not always 30 days per month.
this may be an option to get months between two dates.
$d1 = strtotime("2013-12-09"); $d2 = strtotime("2014-03-17"); echo abs((date('Y', $d2) - date('Y', $d1))*12 + (date('m', $d2) - date('m', $d1))); // 3
Other method to calculate the month difference
$d1 = strtotime("2013-12-09"); $d2 = strtotime("2014-03-17"); $min_date = min($d1, $d2); $max_date = max($d1, $d2); $i = 0; while (($min_date = strtotime("+1 MONTH", $min_date)) <= $max_date) { $i++; } echo $i; // 3
If you want to include remaining days or current month you can add +1 to the result.
Calculate days between two dates:
Using DateTime for PHP >=5.3
$d1 = new DateTime("2013-12-09"); $d2 = new DateTime("2014-03-17"); echo $d1->diff($d2)->days; // 98
Note: But for windows 7 there is a bug, It may always return 6015 as a result for $d1->diff($d2)->days instead of 98.
For PHP < 5.3 you can use timestamps to get days between two dates using strtotime() function.
$d1 = "2013-12-09"; $d2 = "2014-03-17"; $days = floor(abs(strtotime($d2) - strtotime($d1))/(60*60*24)); echo $days. "Days";
You may use below code also to calculate days between dates.
$d1 = strtotime("2013-12-09"); $d2 = strtotime("2014-03-17"); $min_date = min($d1, $d2); $max_date = max($d1, $d2); $i = 0; while (($min_date = strtotime("+1 day", $min_date)) <= $max_date) { $i++; } echo $i; // 98
Conclusion: So there are several different ways to calculate the difference between two dates in PHP which depends on some conditions like PHP version etc. DateTime is the best to calculate the difference if you have >=5.3 else you can use timestamps.
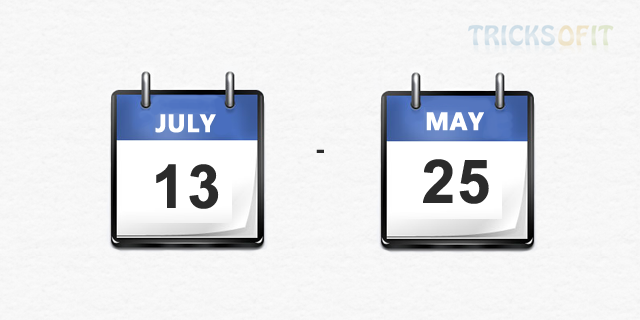