Email is the most popular Internet service nowadays. Every day plenty of mail are sent. In this article I am explaining how to send email in php. Every web application used the email feature as in signup response, monthly newsletter etc. Sometimes email contains a plain text, HTML body and file attachments. I will explain each with a simple example.
Plain Text Email:
PHP has mail() function to send an email. This function requires three mandatory parameters recipient’s email address, subject of email and body of the email. There are other two optional parameters to use.
mail( to, subject, message, headers, parameters );
<?php $to = "someone@somedomain.com"; $subject = "Simple subject"; $message = "This is simple plain text mail."; $header = "From:xyz@somedomain.com \r\n"; if(mail ($to,$subject,$message,$header)){ echo "Email sent successfully"; } else { echo "Email could not be sent"; } ?>
The $to is the recipient email. The $subject is the subject of email. The $message is text content of email. If the mail() function returns true it means mail has been sent.
HTML Email:
If you try to sent email with HTML content as example above, it will be treated as text email When you send a text message using PHP then all the content will be treated as simple text. To send a proper HTML mail you need to specify a Mime version, content type and character set to send an HTML email using PHP.
$to = "someone@somedomain.com"; $subject = "Simple subject"; $message = "This is <b>HTML mail</b>'."; $message .= "<h1>This is the title.</h1>"; $header = "From:xyz@somedomain.com \r\n"; $header .= "Cc:abc@somedomain.com \r\n"; $header .= "MIME-Version: 1.0 \r\n"; $header .= "Content-type: text/html \r\n"; if(mail ($to,$subject,$message,$header)){ echo "Email sent successfully"; } else { echo "Email could not be sent"; }
Attachments with Email:
To send mixed content with email you need to use content-type header to ‘multipart/mixed‘ and text and attachments specifies within boundaries.
$to = "someone@somedomain.com"; $subject = "Simple mail with attachment"; $message = "This is an email with attachment'."; $message .= "<h1>This is the title.</h1>"; $random_hash = md5(date('r', time())); $header = "From:xyz@somedomain.com \r\n"; $header .= "Cc:abc@somedomain.com \r\n"; $header .= "MIME-Version: 1.0 \r\n"; $header .= "Content-Type: multipart/mixed; boundary=\"PHP-mixed-".$random_hash."\""; $attachment = chunk_split(base64_encode(file_get_contents('attachment.txt'))); $msg = 'Content-Type: multipart/mixed; name="attachment.txt" \r\n'; $msg .= 'Content-Transfer-Encoding: base64 \r\n'; $msg .= 'Content-ID: <attachment.txt> \r\n'; $msg .= '\r\n' . $attachment . '\r\n \r\n'; $message .= $msg; $message .= '\r\n' . '--' . $random_hash. '--' . '\r\n'; if(mail ($to,$subject,$message,$header)){ echo "Email sent successfully"; } else { echo "Email could not be sent"; }
Now you must have an idea how the email has been sent with an attachment and html content.
If you have any query you can add comments.
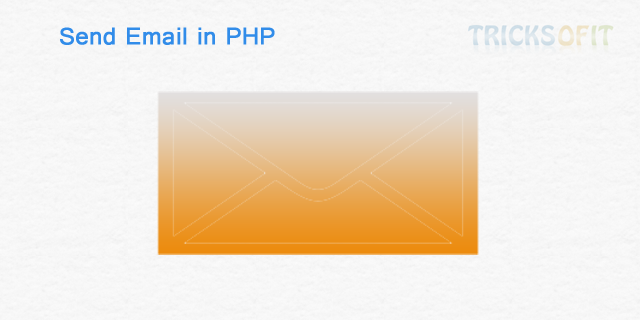