I have published a tutorial on How to Auto Post on Facebook Using PHP with Graph API which post status on user’s wall. Now in this tutorial I am going to show you how to post into a facebook page using Graph API with PHP.

Create Facebook APP:
To get the Facebook Access token you need to create Facebook App. To create a Facebook App please follow the steps of previous post How to Auto Post on Facebook Using PHP and get the App ID and App secret key.
Get Facebook Access Token:
Now to get Facebook Access Token please follow the instruction on my previous tutorial Get Facebook Access Token Using PHP SDK v5.
You need to add the manage_pages, publish_pages and publish_actions permissions to your app. This will let the application access the pages you own, and give the application permission to post to these pages. This permission will give an application access to all the pages you own – not just the one you want to use.
So just change following line
$params = array('req_perms' => 'publish_actions');
To
$params = array('req_perms' => 'publish_actions', 'manage_pages','publish_pages');
Get Page Access Token:
To get page access token create a page getPageAccessToke.php and add following code lines and change as per instructions. This way you will get the access token for your page.
require_once __DIR__ . '/FacebookSDK/src/Facebook/autoload.php'; $fbData = array( 'consumer_key' => APP_ID, 'consumer_secret' => APP_SECRET, 'default_graph_version' => 'v2.2' ); $fb = new Facebook\Facebook($fbData); $page_id = 'your FB page ID'; $accessToken = $accessToken; // that you got from above step $data = $fb->get('/me/accounts', $accessToken); $pages = json_decode($data->getBody()); $page_access_token = ""; foreach($pages->data as $page){ if($page->id == $page_id){ $page_access_token = $page->access_token; break; } } echo $page_access_token; // now you have the access token of the page
Post on Facebook page:
Create a page postOnFbpage.php file and add following code into the file. Now change the fields according to you.
require_once __DIR__ . '/FacebookSDK/src/Facebook/autoload.php'; $fbData = array( 'consumer_key' => APP_ID, 'consumer_secret' => APP_SECRET, 'default_graph_version' => 'v2.2' ); $fb = new Facebook\Facebook($fbData); $page_id = 'your FB page ID'; //// get following data from the db or just replace them //// $params["message"] = '{title}'; $params["link"] = '{targetUrl}'; $params["picture"] = '{imgUrl}'; $params["description"] = '{description}'; // $page_access_token that you get from above step $page_access_token = '{$page_access_token}'; // post to Facebook Page try { $response = $fb->post('/'.$page_id.'/feed', $params, $page_access_token); } catch(Facebook\Exceptions\FacebookResponseException $e) { echo 'Graph returned an error: ' . $e->getMessage(); exit; } catch(Facebook\Exceptions\FacebookSDKException $e) { echo 'Facebook SDK returned an error: ' . $e->getMessage(); exit; } $graphNode = $response->getGraphNode(); echo 'Posted with id: ' . $graphNode['id'];
I have posted following content with above code.
$title = "Post into a Facebook page Using Graph API"; $targetUrl = "http://www.tricksofit.com/2015/10/post-into-a-facebook-page-using-graph-api"; $imgUrl = "http://www.tricksofit.com/wp-content/uploads/2015/10/Post-into-a-facebook-page-Using-Graph-API.png"; $description= "In this tutorial I am going to show you how to post into a facebook page using Graph API with PHP. Get facebook page access token and post on page.";
Ans I got following result on my Facebook page.
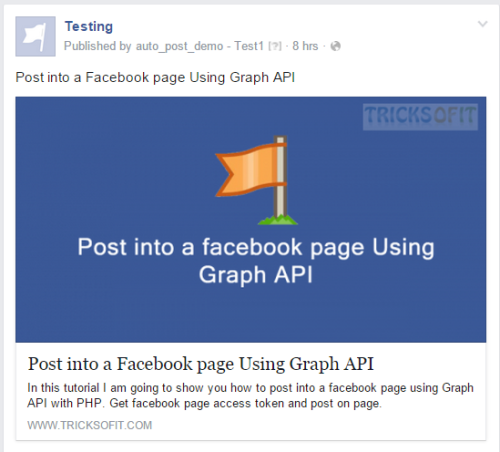
I hope this tutorial will help you to post into a Facebook page Using Graph API.