Now a days Facebook App is used by every other website. These websites shares posts automatically to the users wall using these app and users access token. So today I will show how to auto post on Facebook using PHP.
You have to create a Facebook app and you need a PHP SDK to communicate with the Facebook API. Then you need to get the Access Token, so the app can post on your behalf. After 60 days you need to renew the token because of expiration. I have used a demo app ‘autoÂ_ÂpÂoÂsÂtÂ_Âdemo’ to describe all steps.
Facebook PHP SDK:
Download Facebook PHP SDK (v.3.2.3) from the github (https://github.com/facebookarchive/facebook-php-sdk). This version requires PHP 5.2.0 or higher, PHP curl extension and Curl extension.
This SDK will contain following files:
- facebook.php (main class)
- base_facebook.php (base class)
- fb_ca_chain_bundle.crt (SSL certificate file)
Create Facebook APP:
Go to https://developers.facebook.com/apps and login with your account. Then under Apps menu click on Add a New App and give the app name as you want.

Now your Facebook has been created and you can see App credentials,  App ID and App Secret on dashboard.
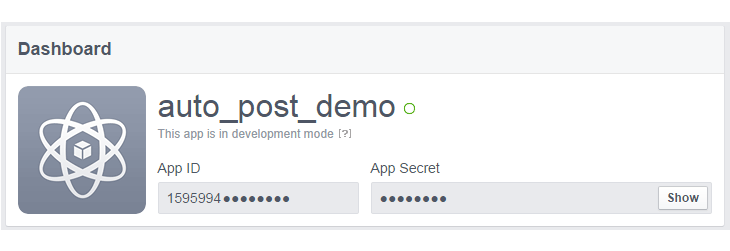
Now go to Settings and then Advanced tab to fill “Deauthorize Callback URL” and “Valid OAuth redirect URIs” and save the changes.
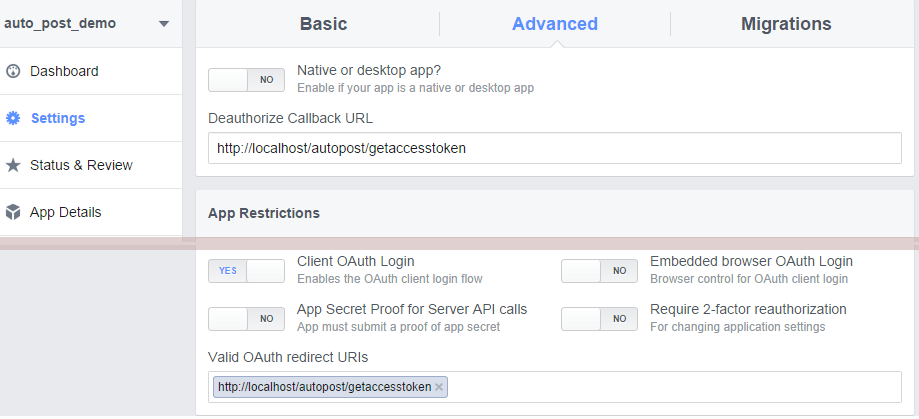
Get Facebook Access Token:
1. Create a callback Page : As I have set “http://localhost/demos/autopost/getaccesstoken/” as a callback URL for the Facebook app, so I have created a index.php file to get the result.
2. Authorize the app for permissions : Read about the facebook permission on https://developers.facebook.com/docs/facebook-login/permissions/v2.2 .
http://www.facebook.com/dialog/oauth?client_id=15960021********&redirect_uri=http://localhost/demos/autopost/&state=458e212e9ee367a9da1060fabc8fe0c4&scope=publish_actions
scope is about Facebook permissions. Here publish_actions is to allow the app to post on user’s Facebook wall.
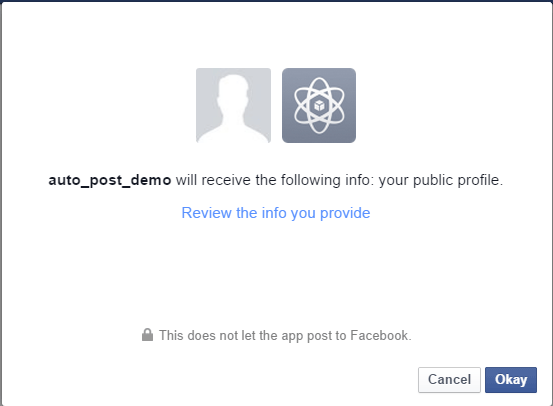
Click Okay button
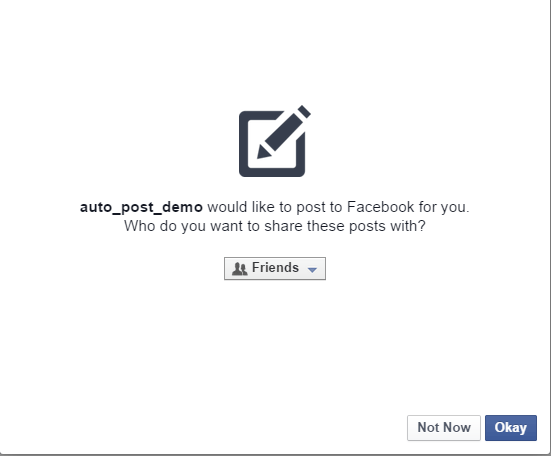
Now you will get the access token as follows:

4. Check your access Token:Â The Facebook access token obtained by the above procedure will be expire after 60 days.
You can get all info about your token from https://developers.facebook.com/tools/debug/accesstoken about expiration and scope.
Renew Facebook Token after expiration:
Facebook Access token expires in 60 days, so you can renew the token by follow step 2-4 as described above.
Now For example share a link with some content to personal profile.
I have created a class FacebookApi to manage connection and share on Facebook with ease.
require_once("FacebookSDK/facebook.php"); class FacebookApi { var $consumer; var $token; var $method; var $http_status; var $last_api_call; var $callback; var $connection; var $access_token; function __construct($data){ $config = array(); $config['appId'] = $data['consumer_key']; $config['secret'] = $data['consumer_secret']; $this->connection = new Facebook($config); } function share($title, $targetUrl, $imgUrl, $description, $access_token){ $this->connection->setAccessToken($access_token); $params["access_token"] = $access_token; if(!empty($title)){ $params["message"] = $title; $params["name"] = $title; } if(!empty($targetUrl)){ $params["link"] = $targetUrl; } if(!empty($imgUrl)){ $params["picture"] = $imgUrl; } if(!empty($description)){ $params["description"] = $description; } // post to Facebook try { $ret = $this->connection->api('/me/feed', 'POST', $params); } catch(Exception $e) { $e->getMessage(); } return true; } function getLoginUrl($params){ return $this->connection->getLoginUrl($params); } function getContent($url) { $ci = curl_init(); /* Curl settings */ curl_setopt($ci, CURLOPT_URL, $url); curl_setopt($ci, CURLOPT_RETURNTRANSFER, true); curl_setopt($ci, CURLOPT_HEADER, false); curl_setopt( $ci, CURLOPT_CONNECTTIMEOUT, 10 ); curl_setopt($ci, CURLOPT_SSL_VERIFYPEER, false); $response = curl_exec($ci); curl_close ($ci); return $response; } }
So you can use this class as follow:
$access_token = 'CAAWrjFLZAK7gBAHmCGmSOCNFh3NMZAI9nrF************'; $facebookData = array(); $facebookData['consumer_key'] = '15960021********'; $facebookData['consumer_secret'] = '3143bb714719b3c***********'; $title = "How to Auto Post on Facebook Using PHP"; $targetUrl = "http://www.tricksofit.com/2015/01/how-to-auto-post-on-facebook-using-php"; $imgUrl = "http://www.tricksofit.com/wp-content/uploads/2015/01/HOW-TO-AUTO-POST-ON-FACEBOOK-USING-PHP.png"; $description = "Every other website shares posts automatically to the user's facebook wall using facebook app. So today I will show how to auto post on Facebook using PHP."; $facebook = new FacebookApi($facebookData); $facebook->share($title, $targetUrl, $imgUrl, $description, $access_token);
Now the post will be display as below on user’s Facebook wall.
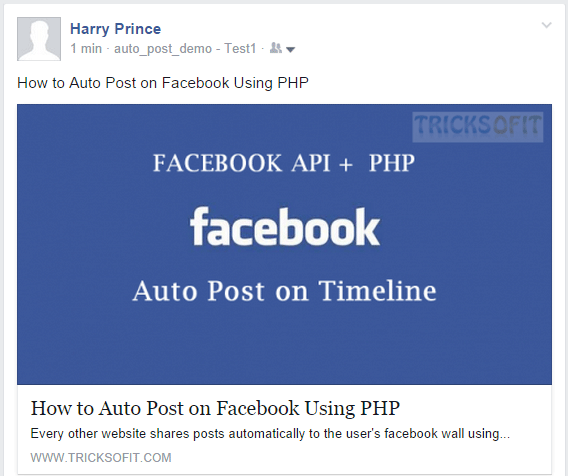
Finally you can store these access token of users in a database.  Now you can create cron script to auto post on user’s Facebook wall.
Please read following tutorials on based on Facebook PHP SDK 5.0