In my previous tutorials I have explained how you can get user access token for Twitter and Facebook. In this tutorial I am sharing, how you can get user access token for LinkedIn.
To get Access Token for LinkedIn you need to create a LinkedIn application and get the Authentication Keys Client ID and Client Secret. Also you will need to use LinkedIn API with OAuth PHP library to get the user Access Token. This Access Token will expire in 60 days.
Create a LinkedIn Application:
To create a LinkedIn application follow following steps:
1. Go to https://www.linkedin.com/developer/apps

2. Now click on Create Application button.

3. Fill required fields in this form and submit. Now your application has been created successfully.
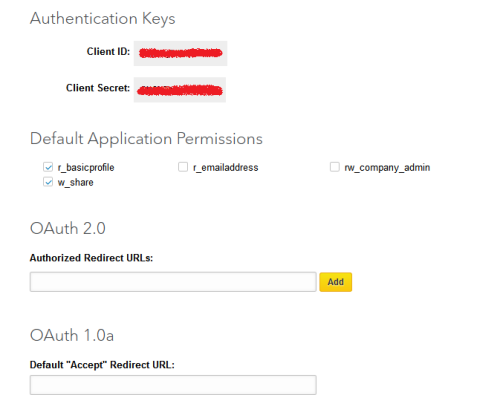
You can get the client ID and client secret under Authentication Keys.
Check w_share in “Default Application Permissions” and update. The w_share permission will grant you the permission to share content on LinkedIn using the API.
Get Access Token:
To get access token you need to use LinkedIn API and OAuth. So download OAuth PHP Library. Now create a file getaccesstoken.php and copy following code:
require_once("OAuth.php"); $data = array( Â Â Â 'consumer_key' => '{CLIENT_ID}', Â Â Â 'consumer_secret' => '{CLIENT_SECRET}', Â Â Â 'callback_url' => 'callback.php' ); $method = new OAuthSignatureMethod_HMAC_SHA1(); $consumer = new OAuthConsumer($data['consumer_key'], $data['consumer_secret']); $token = NULL; $args = array('scope' => 'w_share'); $request = OAuthRequest::from_consumer_and_token($consumer, $token, 'GET', "https://api.linkedin.com/uas/oauth/requestToken", $args); $request->set_parameter("oauth_callback", $data['callback_url']); $request->sign_request($method, $consumer, $token); $request = http($request->to_url()); function http($url, $post_data = null) { $ch = curl_init(); if(defined("CURL_CA_BUNDLE_PATH")) curl_setopt($ch, CURLOPT_CAINFO, CURL_CA_BUNDLE_PATH); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 30); curl_setopt($ch, CURLOPT_TIMEOUT, 30); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0); if(isset($post_data)) { curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, $post_data); } $response = curl_exec($ch); curl_close($ch); return $response; } parse_str($request, $token); $_SESSION['oauth_request_token'] = $token['oauth_token']; $_SESSION['oauth_request_token_secret'] = $token['oauth_token_secret']; if(is_array($token)){ $token = $token['oauth_token']; } $request_link = "https://api.linkedin.com/uas/oauth/authorize?oauth_token=" . $token; header('Location: '. $request_link); exit;
You will be redirected to a LinkedIn page for allow the application to share the content on your behalf. The screen will be like below:

After click on Allow Access you will be redirected to the callback page.
Now create a callback.php page to get the access token.
require_once("OAuth.php"); $data = array( 'consumer_key' => '{CLIENT_ID}', 'consumer_secret' => '{CLIENT_SECRET}', 'callback_url' => 'callback.php' ); if(isset($_REQUEST['oauth_verifier'])){ $data['oauth_token'] = $_SESSION['oauth_request_token']; $data['oauth_token_secret'] = $_SESSION['oauth_request_token_secret']; $method = new OAuthSignatureMethod_HMAC_SHA1(); $consumer = new OAuthConsumer($data['consumer_key'], $data['consumer_secret']); $token = new OAuthConsumer($data['oauth_token'],$data['oauth_token_secret']); $args = array(); $request = OAuthRequest::from_consumer_and_token($consumer, $token, 'GET', "https://api.linkedin.com/uas/oauth/accessToken", $args); $request->set_parameter("oauth_verifier", $_REQUEST['oauth_verifier']); $request->sign_request($method, $consumer, $token); $request = http($request->to_url()); function http($url, $post_data = null) { $ch = curl_init(); if(defined("CURL_CA_BUNDLE_PATH")) curl_setopt($ch, CURLOPT_CAINFO, CURL_CA_BUNDLE_PATH); curl_setopt($ch, CURLOPT_URL, $url); curl_setopt($ch, CURLOPT_CONNECTTIMEOUT, 30); curl_setopt($ch, CURLOPT_TIMEOUT, 30); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, 0); if(isset($post_data)) { curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, $post_data); } $response = curl_exec($ch); curl_close($ch); return $response; } parse_str($request, $token); $tokens = new OAuthConsumer($token['oauth_token'], $token['oauth_token_secret'], 1); $access_token = serialize($tokens); }
You can use this access token to share the content on LinkedIn. Hope this tutorial will help you.